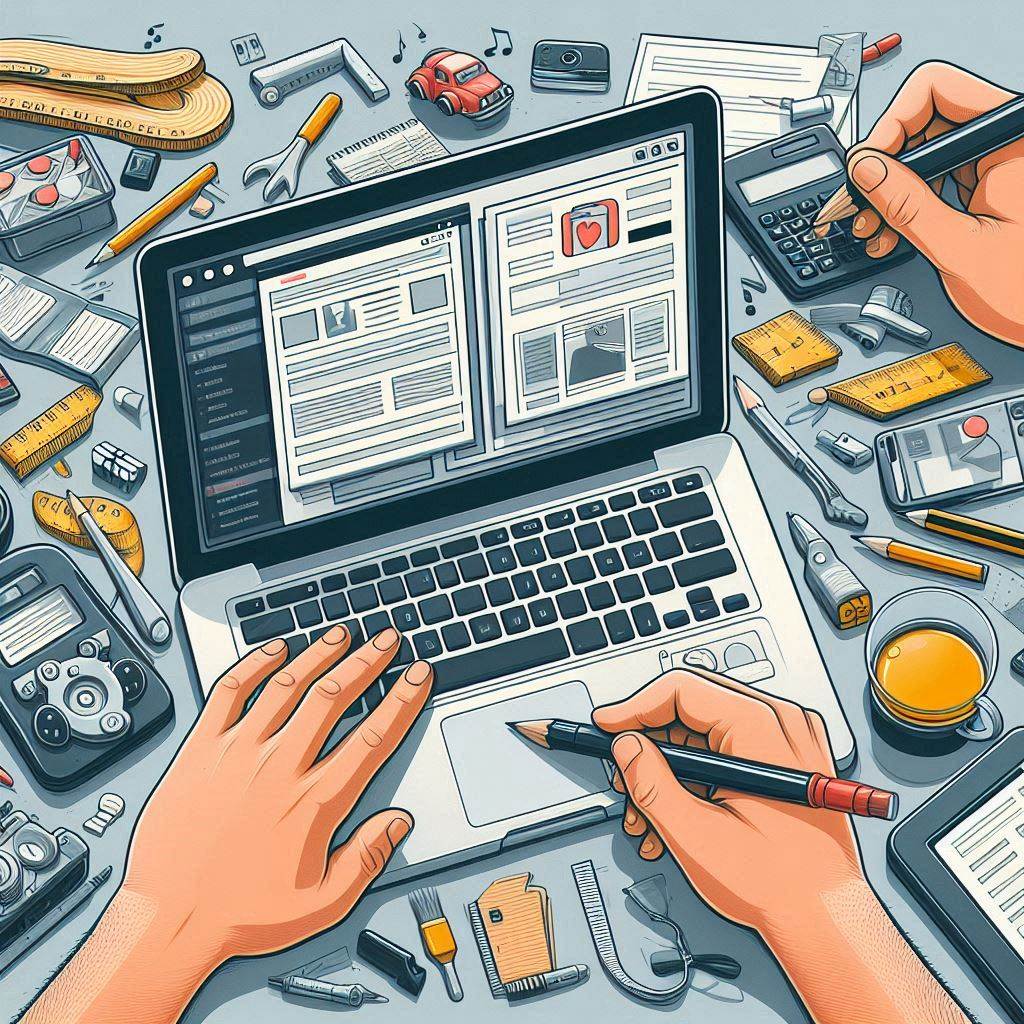
Creating a custom WordPress theme from scratch can be a rewarding experience, giving you full control over the design, functionality, and user experience of your website. It’s important to understand both WordPress’s architecture and best practices in coding to ensure that your theme is efficient, scalable, and easy to maintain. This tutorial will guide you through the process of designing and developing a WordPress theme, covering design principles, template hierarchy, and coding best practices.
Prerequisites
Before you begin, you should have a basic understanding of HTML, CSS, JavaScript, and PHP. You’ll also need:
- A local development environment (e.g., MAMP, WAMP, or XAMPP) or a web server with WordPress installed.
- A code editor (e.g., Visual Studio Code or Sublime Text).
- Basic knowledge of WordPress and its file structure.
1. Understand WordPress Theme Structure
A WordPress theme is a collection of files that work together to present content on your website. The core components of a theme include:
- HTML: Structure of the website.
- CSS: Styling the website.
- JavaScript: Adding dynamic behavior.
- PHP: Connecting to WordPress and using its functions.
The minimum files required for a WordPress theme are:
style.css
: This is the main stylesheet for the theme.index.php
: The default template file for displaying content.functions.php
: This file contains theme functions and hooks.
2. Setting Up Your Theme
Start by creating a new folder for your theme in the /wp-content/themes/
directory of your WordPress installation. Name it according to your theme, for example, my-custom-theme
. Inside this folder, create the following files:
style.css
This file not only contains the CSS for your theme but also has important metadata about your theme in the form of comments. Add the following at the top of style.css
:
/*
Theme Name: My Custom Theme
Theme URI: http://yourwebsite.com/
Author: Your Name
Author URI: http://yourwebsite.com/
Description: A custom WordPress theme built from scratch.
Version: 1.0
*/
index.php
This is the main template file, which WordPress uses as the fallback to display content if no other template is available. For now, add a simple HTML structure:
<!DOCTYPE html>
<html <?php language_attributes(); ?>>
<head>
<meta charset="<?php bloginfo('charset'); ?>">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title><?php bloginfo('name'); ?></title>
<link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>">
</head>
<body>
<header>
<h1><?php bloginfo('name'); ?></h1>
<p><?php bloginfo('description'); ?></p>
</header>
<main><?php
if (have_posts()) :
while (have_posts()) : the_post();
the_content();
endwhile;
else :
echo ‘<p>No content found</p>’;
endif;
?>
</main>
<footer><p>© <?php echo date(‘Y’); ?> <?php bloginfo(‘name’); ?></p>
</footer>
</body>
</html>
functions.php
The functions.php
file is used to add theme support, enqueue scripts, and set up various functionalities. Start by adding this code to enable WordPress features:
<?php
// Enqueue styles and scripts
function my_custom_theme_scripts() {
wp_enqueue_style('style', get_stylesheet_uri());
}
add_action(‘wp_enqueue_scripts’, ‘my_custom_theme_scripts’);
// Add theme support for title tagadd_theme_support(‘title-tag’);
// Add theme support for post thumbnails
add_theme_support(‘post-thumbnails’);
// Register a custom navigation menu
function my_custom_theme_menus() {
register_nav_menus(array(
‘primary’ => __(‘Primary Menu’, ‘my-custom-theme’),
));
}
add_action(‘init’, ‘my_custom_theme_menus’);
?>
3. Template Hierarchy
WordPress uses a specific template hierarchy to decide which file to load when displaying a page. It starts at the most specific template and falls back to more general ones. Understanding this hierarchy allows you to create templates for specific purposes. Here are some key template files you might create:
single.php
: Used to display individual posts.page.php
: Displays static pages.archive.php
: Displays post archives, categories, or tag listings.header.php
: Contains the site’s header, usually with the logo, title, and navigation.footer.php
: Contains the footer section.sidebar.php
: Displays the sidebar content.404.php
: Displays a custom 404 error page.
For example, you can create a header.php
file and include it in your other templates with the following code in index.php
:
<?php get_header(); ?>
And similarly for the footer:
<?php get_footer(); ?>
This way, the header and footer can be reused across multiple templates.
4. Design Principles
When designing your theme, keep the following principles in mind:
- Consistency: Maintain a consistent design throughout your website. Use the same color scheme, typography, and layout structure.
- User Experience: Ensure your website is easy to navigate. Use clear labels, simple navigation menus, and well-organized content.
- Mobile Responsiveness: Make sure your design works well on all screen sizes. Use media queries in your CSS to adjust layouts for mobile and tablet users.
- Accessibility: Design with accessibility in mind, ensuring that all users can access and navigate your site. Use proper heading structures and alt text for images.
5. Best Practices for Coding
a) Use WordPress Functions and Hooks
WordPress provides a wide range of built-in functions to help you work with content, users, and more. For example, use the_title()
and the_content()
to display post titles and content. Always try to use WordPress functions rather than hardcoding elements.
b) Keep Code Modular
Split your theme into reusable components like header.php
, footer.php
, and sidebar.php
. This keeps your code modular and easier to maintain.
c) Security
Sanitize all user inputs to avoid vulnerabilities like Cross-Site Scripting (XSS). Use functions like esc_html()
, esc_url()
, and wp_kses_post()
when outputting data.
d) Enqueue Scripts and Styles
Always use wp_enqueue_script()
and wp_enqueue_style()
to load JavaScript and CSS files rather than hardcoding them into your theme. This ensures compatibility with plugins and avoids conflicts.
For example:
function my_custom_theme_enqueue_scripts() {
wp_enqueue_style('main-style', get_stylesheet_uri());
wp_enqueue_script('main-script', get_template_directory_uri() . '/js/main.js', array(), false, true);
}
add_action('wp_enqueue_scripts', 'my_custom_theme_enqueue_scripts');
e) Use Template Tags
WordPress has many template tags, like the_post_thumbnail()
for images and the_permalink()
for post links, which simplify theme development and improve readability.
6. Customizing Your Theme
Once you have the basic structure in place, you can begin to add customizations:
- Custom Post Types: If your website has unique content types, like portfolios or testimonials, consider creating custom post types in
functions.php
. - Custom Widgets: You can register widget areas for sidebars or footers using
register_sidebar()
. - Theme Customizer: Use the WordPress Customizer API to allow users to change theme settings from the WordPress admin area.
7. Testing and Debugging
Before launching your theme, thoroughly test it on various devices and browsers. Use tools like the WordPress Theme Unit Test Data to ensure your theme displays content consistently. Debugging tools like WP_DEBUG
can help catch errors in your theme.
Creating a custom WordPress theme from scratch gives you full control over your website’s appearance and functionality. By understanding WordPress’s template hierarchy, coding best practices, and design principles, you can build a theme that is both visually appealing and easy to manage. Keep experimenting, testing, and refining your theme, and soon you’ll have a polished, custom WordPress theme tailored to your needs.